开源 AI 食谱文档
使用 Spaces 和 Gradio 创建演示
并获得增强的文档体验
开始使用
使用 Spaces 和 Gradio 创建演示
简介
在本笔记本中,我们将演示如何使用 Gradio 将任何机器学习模型变为现实。Gradio 是一个库,允许您从任何 Python 函数创建 Web 演示,并与世界 🌎 分享!
📚 本笔记本涵盖了
- 构建
Hello, World!
演示:Gradio 的基础知识 - 将您的演示移至 Hugging Face Spaces
- 使其有趣:利用 🤗 Hub 的真实世界示例
- Gradio 附带的一些很酷的“内置”功能
⏭️ 在本笔记本的末尾,您将找到一个 Further Reading
列表,其中包含继续自主学习的链接。
设置
首先,安装 gradio
库以及 transformers
。
!pip -q install gradio==4.36.1
!pip -q install transformers==4.41.2
# the usual shorthand is to import gradio as gr
import gradio as gr
您的第一个演示:Gradio 的基础知识
Gradio 的核心是将任何 Python 函数转换为 Web 界面。
假设我们有一个简单的函数,它接受 name
和 intensity
作为参数,并返回如下字符串
def greet(name: str, intensity: int) -> str:
return "Hello, " + name + "!" * int(intensity)
如果您对名称 ‘Diego’ 运行此函数,您将获得如下所示的输出字符串
>>> print(greet("Diego", 3))
Hello, Diego!!!
使用 Gradio,我们可以通过 gr.Interface
类为此函数构建一个界面。我们只需要传入上面创建的 greet
函数,以及我们的函数期望的输入和输出类型
demo = gr.Interface(
fn=greet,
inputs=["text", "slider"], # the inputs are a text box and a slider ("text" and "slider" are components in Gradio)
outputs=["text"], # the output is a text box
)
请注意,我们如何传入 ["text", "slider"]
作为输入,["text"]
作为输出 —— 这些在 Gradio 中被称为 组件 (Components)。
这就是我们第一个演示所需的一切。继续尝试一下 👇🏼!在 name
文本框中输入您的姓名,滑动您想要的强度,然后单击 Submit
。
# the launch method will fire up the interface we just created
demo.launch()
让它变得有趣:会议转录工具
此时,您已经了解了如何将基本的 Python 函数转换为可用于 Web 的演示。但是,我们只对一个非常简单,甚至有点无聊的函数执行了此操作!
让我们考虑一个更有趣的示例,它突出了 Gradio 构建的真正目的:演示最前沿的机器学习模型。我的一位好朋友最近请我帮忙处理她做的一次采访的录音。她需要将音频文件转换为组织良好的文本摘要。我是如何帮助她的呢?我构建了一个 Gradio 应用程序!
让我们逐步了解构建会议转录工具的步骤。我们可以将该过程分为两个部分
- 将音频文件转录为文本
- 将文本组织成章节、段落、列表等。我们也可以在此处包含摘要。
音频转文本
在这一部分,我们将构建一个演示,处理会议转录工具的第一步:将音频转换为文本。
正如我们所了解的,构建 Gradio 演示的关键在于拥有一个 Python 函数来执行我们想要展示的逻辑。对于音频转文本的转换,我们将使用出色的 transformers
库及其 pipeline
实用程序来构建我们的函数,以使用流行的音频转文本模型,称为 distil-whisper/distil-large-v3
。
结果是以下 transcribe
函数,它将我们要转换的音频作为输入
import os
import tempfile
import torch
import gradio as gr
from transformers import pipeline
device = 0 if torch.cuda.is_available() else "cpu"
AUDIO_MODEL_NAME = (
"distil-whisper/distil-large-v3" # faster and very close in performance to the full-size "openai/whisper-large-v3"
)
BATCH_SIZE = 8
pipe = pipeline(
task="automatic-speech-recognition",
model=AUDIO_MODEL_NAME,
chunk_length_s=30,
device=device,
)
def transcribe(audio_input):
"""Function to convert audio to text."""
if audio_input is None:
raise gr.Error("No audio file submitted!")
output = pipe(audio_input, batch_size=BATCH_SIZE, generate_kwargs={"task": "transcribe"}, return_timestamps=True)
return output["text"]
现在我们有了 Python 函数,我们可以通过将其传递到 gr.Interface
中来演示它。请注意,在这种情况下,该函数期望的输入是我们想要转换的音频。Gradio 包含大量有用的组件,其中之一是 Audio,这正是我们演示所需的 🎶 😎。
part_1_demo = gr.Interface(
fn=transcribe,
inputs=gr.Audio(type="filepath"), # "filepath" passes a str path to a temporary file containing the audio
outputs=gr.Textbox(show_copy_button=True), # give users the option to copy the results
title="Transcribe Audio to Text", # give our demo a title :)
)
part_1_demo.launch()
继续尝试一下 👆!您可以上传 .mp3
文件或点击 🎤 按钮录制自己的声音。
对于包含实际会议录音的示例文件,您可以查看 MeetingBank_Audio 数据集,这是一个来自美国 6 个主要城市市议会的会议数据集。为了进行我自己的测试,我尝试了几个 丹佛会议。
另请查看 Interface
的 from_pipeline 构造函数,它将直接从 pipeline
构建 Interface
。
组织 & 总结文本
对于会议转录工具的第二部分,我们需要组织上一步转录的文本。
再一次,为了构建 Gradio 演示,我们需要具有我们关心的逻辑的 Python 函数。对于文本组织和摘要,我们将使用“指令调整 (instruction-tuned)” 模型,该模型经过训练可以执行各种任务。有很多选项可供选择,例如 meta-llama/Meta-Llama-3-8B-Instruct 或 mistralai/Mistral-7B-Instruct-v0.3。在我们的示例中,我们将使用 microsoft/Phi-3-mini-4k-instruct。
就像第 1 部分一样,我们可以利用 transformers
中的 pipeline
实用程序来完成此操作,但相反,我们将借此机会展示 无服务器推理 API (Serverless Inference API),它是 Hugging Face Hub 中的一个 API,允许我们免费使用数千个公开可访问(或您自己的私有权限)的机器学习模型!查看此处无服务器推理 API 的 Cookbook 部分:此处。
使用无服务器推理 API 意味着我们将从 InferenceClient
调用模型,而不是像音频转换部分那样通过 pipeline 调用模型。InferenceClient
是 huggingface_hub
库的一部分(Hub Python 库)。反过来,要使用 InferenceClient
,我们需要使用 notebook_login()
登录到 🤗 Hub,这将弹出一个对话框,要求您提供用户访问令牌 (User Access Token) 以向 Hub 进行身份验证。
您可以从您的个人设置页面管理您的令牌,请记住尽可能多地使用细粒度 (fine-grained)令牌以增强安全性。
from huggingface_hub import notebook_login, InferenceClient
# running this will prompt you to enter your Hugging Face credentials
notebook_login()
现在我们已登录到 Hub,我们可以使用无服务器推理 API 通过 InferenceClient
编写我们的文本处理函数。
这部分的代码将构建为两个函数
build_messages
,将消息提示格式化为 LLM;organize_text
,实际将原始会议文本传递到 LLM 以进行组织(和摘要,具体取决于我们提供的提示)。
# sample meeting transcript from huuuyeah/MeetingBank_Audio
# this is just a copy-paste from the output of part 1 using one of the Denver meetings
sample_transcript = """
Good evening. Welcome to the Denver City Council meeting of Monday, May 8, 2017. My name is Kelly Velez. I'm your Council Secretary. According to our rules of procedure, when our Council President, Albus Brooks, and Council President Pro Tem, JoLynn Clark, are both absent, the Council Secretary calls the meeting to order. Please rise and join Councilman Herndon in the Pledge of Allegiance. Madam Secretary, roll call. Roll call. Here. Mark. Espinosa. Here. Platt. Delmar. Here. Here. Here. Here. We have five members present. There is not a quorum this evening. Many of the council members are participating in an urban exploration trip in Portland, Oregon, pursuant to Section 3.3.4 of the city charter. Because there is not a quorum of seven council members present, all of tonight's business will move to next week, to Monday, May 15th. Seeing no other business before this body except to wish Councilwoman Keniche a very happy birthday this meeting is adjourned Thank you. A standard model and an energy efficient model likely will be returned to you in energy savings many times during its lifespan. Now, what size do you need? Air conditioners are not a one-size-or-type fits all. Before you buy an air conditioner, you need to consider the size of your home and the cost to operate the unit per hour. Do you want a room air conditioner, which costs less but cools a smaller area, or do you want a central air conditioner, which cools your entire house but costs more? Do your homework. Now, let's discuss evaporative coolers. In low humidity areas, evaporating water into the air provides a natural and energy efficient means of cooling. Evaporative coolers, also called swamp coolers, cool outdoor air by passing it over water saturated pads, causing the water to evaporate into it. Evaporative coolers cost about one half as much to install as central air conditioners and use about one-quarter as much energy. However, they require more frequent maintenance than refrigerated air conditioners, and they're suitable only for areas with low humidity. Watch the maintenance tips at the end of this segment to learn more. And finally, fans. When air moves around in your home, it creates a wind chill effect. A mere two-mile-an-hour breeze will make your home feel four degrees cooler and therefore you can set your thermostat a bit higher. Ceiling fans and portable oscillating fans are cheap to run and they make your house feel cooler. You can also install a whole house fan to draw the hot air out of your home. A whole house fan draws cool outdoor air inside through open windows and exhausts hot room air through the attic to the outside. The result is excellent ventilation, lower indoor temperatures, and improved evaporative cooling. But remember, there are many low-cost, no-cost ways that you can keep your home cool. You should focus on these long before you turn on your AC or even before you purchase an AC. But if you are going to purchase a new cooling system, remember to get one that's energy efficient and the correct size for your home. Wait, wait, don't go away, there's more. After this segment of the presentation is over, you're going to be given the option to view maintenance tips about air conditioners and evaporative coolers. Now all of these tips are brought to you by the people at Xcel Energy. Thanks for watching.
"""
from huggingface_hub import InferenceClient
TEXT_MODEL_NAME = "microsoft/Phi-3-mini-4k-instruct"
client = InferenceClient()
def organize_text(meeting_transcript):
messages = build_messages(meeting_transcript)
response = client.chat_completion(messages, model=TEXT_MODEL_NAME, max_tokens=250, seed=430)
return response.choices[0].message.content
def build_messages(meeting_transcript) -> list:
system_input = "You are an assitant that organizes meeting minutes."
user_input = """Take this raw meeting transcript and return an organized version.
Here is the transcript:
{meeting_transcript}
""".format(
meeting_transcript=meeting_transcript
)
messages = [
{"role": "system", "content": system_input},
{"role": "user", "content": user_input},
]
return messages
现在我们有了文本组织函数 organize_text
,我们也可以为其构建一个演示
part_2_demo = gr.Interface(
fn=organize_text,
inputs=gr.Textbox(value=sample_transcript),
outputs=gr.Textbox(show_copy_button=True),
title="Clean Up Transcript Text",
)
part_2_demo.launch()
继续尝试一下 👆!如果您在上面的演示中点击“Submit”,您将看到输出文本是转录的更清晰和更有条理的版本,其中包含标题和会议不同部分的章节。
看看您是否可以通过调整控制 LLM 提示的 user_input
变量来获得摘要。
将它们放在一起
此时,我们为会议转录工具要执行的两个步骤中的每一个都提供了一个函数
- 将音频转换为文本文件,以及
- 将该文本文件组织成格式良好的会议文档。
我们接下来要做的就是将这两个函数拼接在一起,并为组合步骤构建一个演示。换句话说,我们完整的会议转录工具只是一个新函数(我们将创造性地称之为 meeting_transcript_tool
😀),它接受 transcribe
的输出并将其传递给 organize_text
def meeting_transcript_tool(audio_input):
meeting_text = transcribe(audio_input)
organized_text = organize_text(meeting_text)
return organized_text
full_demo = gr.Interface(
fn=meeting_transcript_tool,
inputs=gr.Audio(type="filepath"),
outputs=gr.Textbox(show_copy_button=True),
title="The Complete Meeting Transcription Tool",
)
full_demo.launch()
继续尝试一下 👆!这现在是我们转录工具的完整演示。如果您给它一个音频文件,输出将是会议的已组织(并可能已摘要)版本。超级酷 😎。
将您的演示移至 🤗 Spaces
如果您走到这一步,现在您就了解了如何使用 Gradio 创建机器学习模型的演示 👏!
接下来,我们将向您展示如何将您的全新演示移至 Hugging Face Spaces。除了 Gradio 的易用性和强大功能外,将您的演示移至 🤗 Spaces 还可以为您带来永久托管、每次更新应用程序时易于部署以及与任何人分享您的工作的能力!请记住,除非您正在使用或更改 Space,否则您的 Space 会在一段时间后进入休眠状态。
第一步是访问 https://huggingface.co/new-space,从模板中选择“Gradio”,并将其余选项保留为默认值(您可以稍后更改这些选项)
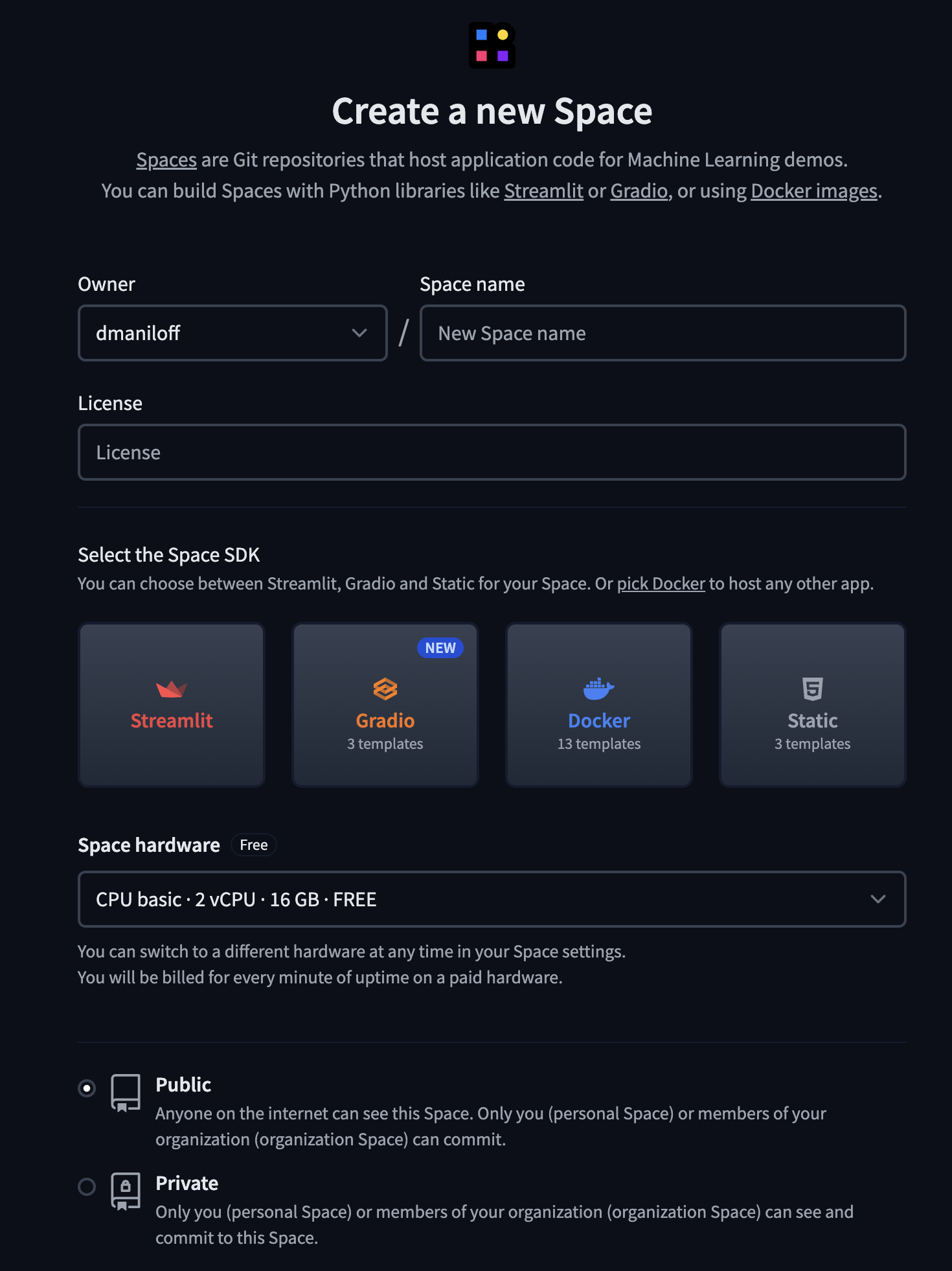
这将创建一个新创建的 Space,您可以在其中填充演示代码。作为供您参考的示例,我创建了 🤗 Space dmaniloff/meeting-transcript-tool
,您可以在此处访问。
我们需要编辑两个文件
app.py
—— 这是演示代码所在的位置。它应该看起来像这样# outline of app.py: def meeting_transcript_tool(...): ... def transcribe(...): ... def organize_text(...): ...
requirements.txt
—— 这是我们告诉 Space 它需要的库的地方。它应该看起来像这样# contents of requirements.txt: torch transformers
Gradio 自带电池 🔋
Gradio 具有许多开箱即用的很酷的功能。我们无法在本笔记本中涵盖所有功能,但以下是我们将介绍的 3 个功能
- 作为 API 访问
- 通过公共 URL 共享
- 标记 (Flagging)
作为 API 访问
使用 Gradio 构建 Web 演示的好处之一是您会自动获得 API 🙌!这意味着您可以使用标准的 HTTP 客户端(如 curl
或 Python requests
库)访问您的 Python 函数的功能。
如果您仔细查看我们上面创建的演示,您将在底部看到一个链接,上面写着“Use via API”。如果您点击我在 Space 中创建的链接 (dmaniloff/meeting-transcript-tool),您将看到以下内容
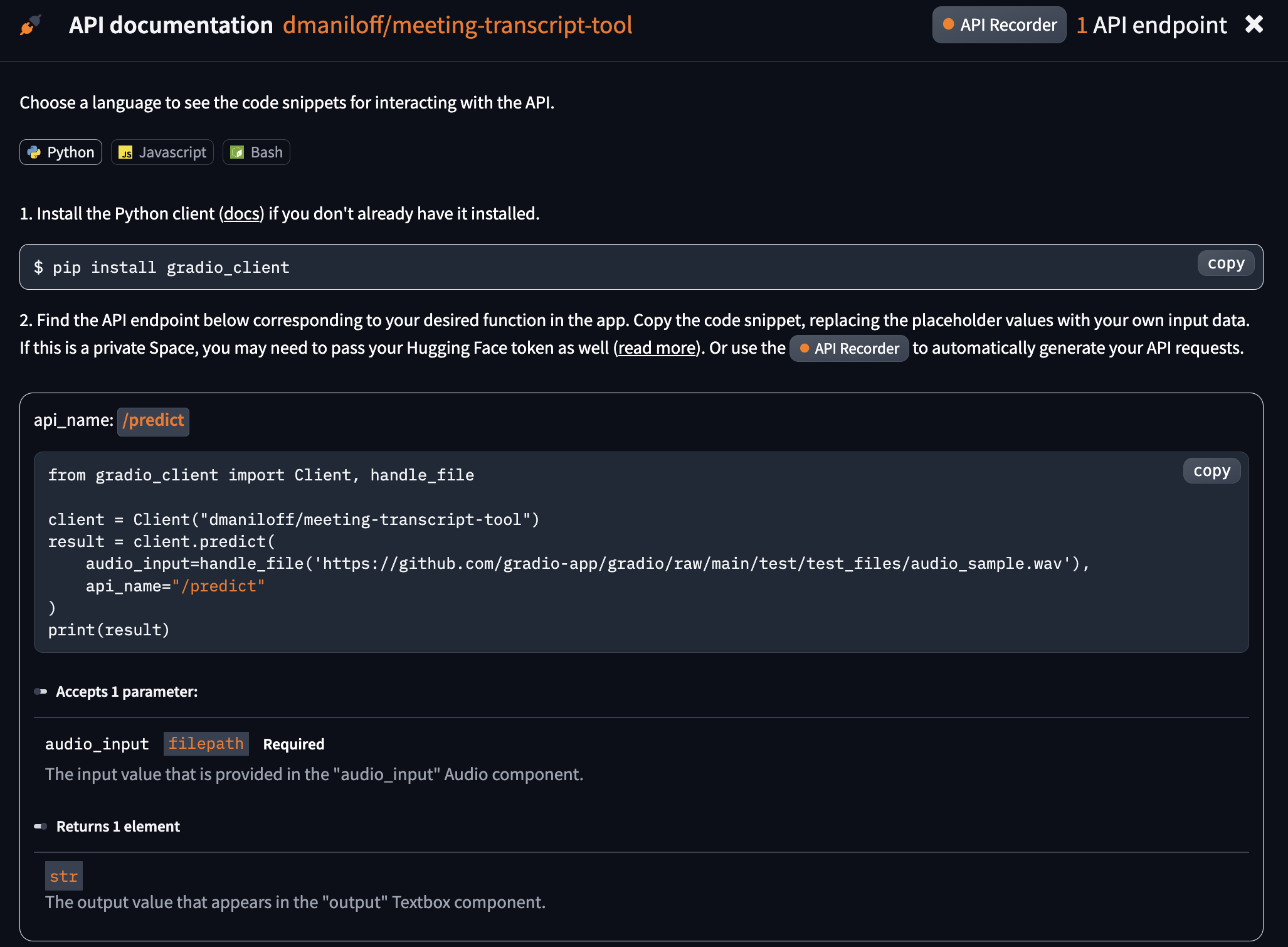
让我们继续复制粘贴下面的代码,将我们的 Space 用作 API
!pip install gradio_client
>>> from gradio_client import Client, handle_file
>>> client = Client("dmaniloff/meeting-transcript-tool")
>>> result = client.predict(
... audio_input=handle_file("https://github.com/gradio-app/gradio/raw/main/test/test_files/audio_sample.wav"),
... api_name="/predict",
... )
>>> print(result)
Loaded as API: https://dmaniloff-meeting-transcript-tool.hf.space ✔ Certainly! Below is an organized version of a hypothetical meeting transcript. Since the original transcript you've provided is quite minimal, I'll create a more detailed and structured example featuring a meeting summary. --- # Meeting Transcript: Project Alpha Kickoff **Date:** April 7, 2023 **Location:** Conference Room B, TechCorp Headquarters **Attendees:** - John Smith (Project Manager) - Emily Johnson (Lead Developer) - Michael Brown (Marketing Lead) - Lisa Green (Design Lead) **Meeting Duration:** 1 hour 30 minutes ## Opening Remarks **John Smith:** Good morning everyone, and thank you for joining this kickoff meeting for Project Alpha. Today, we'll discuss our project vision, milestones, and roles. Let's get started. ## Vision and Goals **Emily Johnson:** The main goal of Project Alpha is to
哇!那里发生了什么?让我们分解一下
- 我们安装了
gradio_client
,这是一个专门设计用于与使用 Gradio 构建的 API 交互的软件包。 - 我们通过提供我们要查询的 🤗 Space 的名称来实例化客户端。
- 我们调用了客户端的
predict
方法,并向其传递了一个示例音频文件。
Gradio 客户端负责为我们发出 HTTP POST 请求,并且还提供读取我们的会议转录工具将处理的输入音频文件(通过函数 handle_file
)等功能。
同样,使用此客户端是一种选择,您也可以运行 curl -X POST https://dmaniloff-meeting-transcript-tool.hf.space/call/predict [...]
并在请求中传递所有需要的参数。
我们从上面的调用中获得的输出是由我们用于文本组织的 LLM 生成的虚构会议。这是因为示例输入文件不是实际的会议录音。您可以调整 LLM 的提示来处理这种情况。
通过公共 URL 共享
Gradio 内置的另一个很酷的功能是,即使您在本地计算机上构建演示(在将其移至 🤗 Space 之前),您仍然可以通过将 share=True
传递到 launch
中来与世界上的任何人共享它,如下所示
demo.launch(share=True)
您可能已经注意到,在此 Google Colab 环境中,默认情况下启用了此行为,因此我们之前创建的演示已经有一个您可以共享的公共 URL 🌎。返回 ⬆ 并查看 Running on public URL:
的日志以找到它 🔎!
标记 (Flagging)
标记 (Flagging) 是 Gradio 内置的一项功能,允许您的演示用户提供反馈。您可能已经注意到,我们创建的第一个演示的底部有一个 Flag
按钮。
在默认选项下,如果用户单击该按钮,则输入和输出样本将保存到 CSV 日志文件中,您可以在以后查看。如果演示涉及音频(就像我们的情况一样),这些音频将单独保存在并行目录中,并且这些文件的路径将保存在 CSV 文件中。
返回并再次尝试我们的第一个演示,然后单击 Flag
按钮。您将看到在 flagged
目录中创建了一个新的日志文件
>>> !cat flagged/log.csv
name,intensity,output,flag,username,timestamp Diego,4,"Hello, Diego!!!!",,,2024-06-29 22:07:50.242707
在本例中,我将输入设置为 name=diego
和 intensity=29
,然后我将其标记。您可以看到日志文件包含函数的输入、输出 "Hello, diego!!!!!!!!!!!!!!!!!!!!!!!!!!!!!"
以及时间戳。
虽然用户发现有问题的输入和输出列表总比没有好,但 Gradio 的标记功能允许您做更多的事情。例如,您可以提供一个 flagging_options
参数,让您自定义可以收到的反馈或错误类型,例如 ["Incorrect", "Ambiguous"]
。请注意,这需要将 allow_flagging
设置为 "manual"
demo_with_custom_flagging = gr.Interface(
fn=greet,
inputs=["text", "slider"], # the inputs are a text box and a slider ("text" and "slider" are components in Gradio)
outputs=["text"], # the output is a text box
allow_flagging="manual",
flagging_options=["Incorrect", "Ambiguous"],
)
demo_with_custom_flagging.launch()
继续尝试一下 👆!您可以看到标记按钮现在是 Flag as Incorrect
和 Flag as Ambiguous
,新的日志文件将反映这些选项
>>> !cat flagged/log.csv
name,intensity,output,flag,username,timestamp Diego,4,"Hello, Diego!!!!",,,2024-06-29 22:07:50.242707 Diego,5,"Hello, Diego!!!!!",Ambiguous,,2024-06-29 22:08:04.281030
总结 & 后续步骤
在本笔记本中,我们学习了如何使用 Gradio 演示任何机器学习模型。
首先,我们学习了为简单的 Python 函数设置界面的基础知识;其次,我们介绍了 Gradio 的真正优势:为机器学习模型构建演示。
为此,我们学习了通过 transformers
库及其 pipeline
函数,以及如何使用 gr.Audio
等多媒体输入,来轻松利用 🤗 Hub 中的模型。
第三,我们介绍了如何在 🤗 Spaces 上托管您的 Gradio 演示,这使您可以让演示在云中运行,并为您提供演示计算要求的灵活性。
最后,我们展示了 Gradio 附带的一些超级酷的内置功能,例如 API 访问、公共 URL 和标记 (Flagging)。
对于后续步骤,请查看每个部分末尾的 Further Reading
链接。
⏭️ 进一步阅读
- 您的第一个 gradio 演示
- Gradio 组件 (Components)
- transformers 库
- pipeline 函数
- Hub Python 库
- 无服务器推理 API (Serverless Inference API)
- 🤗 Spaces
- Spaces 文档