了解管道、模型和调度器
🧨 Diffusers 被设计为一个用户友好且灵活的工具箱,用于构建针对您的用例量身定制的扩散系统。工具箱的核心是模型和调度器。虽然 DiffusionPipeline 将这些组件捆绑在一起以方便起见,但您也可以将管道解绑,并分别使用模型和调度器来创建新的扩散系统。
在本教程中,您将学习如何使用模型和调度器组装一个用于推理的扩散系统,从一个基本管道开始,然后逐步发展到 Stable Diffusion 管道。
分解一个基本管道
管道是一种快速便捷的运行模型以进行推理的方式,只需要四行代码即可生成图像
>>> from diffusers import DDPMPipeline
>>> ddpm = DDPMPipeline.from_pretrained("google/ddpm-cat-256", use_safetensors=True).to("cuda")
>>> image = ddpm(num_inference_steps=25).images[0]
>>> image

这超级简单,但管道是怎么做到的呢?让我们分解管道,看看它内部发生了什么。
在上面的示例中,管道包含一个 UNet2DModel 模型和一个 DDPMScheduler。管道通过将与所需输出大小相同的随机噪声多次传递给模型来对图像进行去噪。在每个时间步长,模型都会预测噪声残差,调度器会使用它来预测一个噪声较小的图像。管道会重复此过程,直到它到达指定的推理步长数的末尾。
要使用模型和调度器分别重建管道,让我们编写自己的去噪过程。
- 加载模型和调度器
>>> from diffusers import DDPMScheduler, UNet2DModel
>>> scheduler = DDPMScheduler.from_pretrained("google/ddpm-cat-256")
>>> model = UNet2DModel.from_pretrained("google/ddpm-cat-256", use_safetensors=True).to("cuda")
- 设置运行去噪过程的时间步长数
>>> scheduler.set_timesteps(50)
- 设置调度器时间步长会在其中创建一个具有均匀间隔元素的张量,在本例中为 50 个。每个元素对应于模型对图像进行去噪的时间步长。当您稍后创建去噪循环时,您将遍历此张量来对图像进行去噪
>>> scheduler.timesteps
tensor([980, 960, 940, 920, 900, 880, 860, 840, 820, 800, 780, 760, 740, 720,
700, 680, 660, 640, 620, 600, 580, 560, 540, 520, 500, 480, 460, 440,
420, 400, 380, 360, 340, 320, 300, 280, 260, 240, 220, 200, 180, 160,
140, 120, 100, 80, 60, 40, 20, 0])
- 创建一些与所需输出形状相同的随机噪声
>>> import torch
>>> sample_size = model.config.sample_size
>>> noise = torch.randn((1, 3, sample_size, sample_size), device="cuda")
- 现在编写一个循环来遍历时间步长。在每个时间步长,模型都会执行 UNet2DModel.forward() 传递并返回噪声残差。调度器的 step() 方法会获取噪声残差、时间步长和输入,并预测前一个时间步长的图像。此输出将成为去噪循环中模型的下一个输入,它将重复,直到它到达
timesteps
数组的末尾。
>>> input = noise
>>> for t in scheduler.timesteps:
... with torch.no_grad():
... noisy_residual = model(input, t).sample
... previous_noisy_sample = scheduler.step(noisy_residual, t, input).prev_sample
... input = previous_noisy_sample
这就是整个去噪过程,您可以使用相同的模式来编写任何扩散系统。
- 最后一步是将去噪后的输出转换为图像
>>> from PIL import Image
>>> import numpy as np
>>> image = (input / 2 + 0.5).clamp(0, 1).squeeze()
>>> image = (image.permute(1, 2, 0) * 255).round().to(torch.uint8).cpu().numpy()
>>> image = Image.fromarray(image)
>>> image
在下一节中,您将测试您的技能,并分解更复杂的 Stable Diffusion 管道。步骤或多或少相同。您将初始化必要的组件,并设置时间步长数以创建一个timestep
数组。timestep
数组用于去噪循环中,并且对于此数组中的每个元素,模型都会预测一个噪声较小的图像。去噪循环会遍历timestep
,并在每个时间步长,它会输出一个噪声残差,调度器会使用它来预测前一个时间步长的噪声较小的图像。重复此过程,直到您到达timestep
数组的末尾。
让我们试试吧!
分解 Stable Diffusion 管道
Stable Diffusion 是一种文本到图像潜在扩散模型。它被称为潜在扩散模型,因为它使用图像的低维表示而不是实际像素空间,这使得它更加节省内存。编码器将图像压缩成更小的表示,解码器将压缩后的表示转换回图像。对于文本到图像模型,您将需要一个标记器和一个编码器来生成文本嵌入。从前面的示例中,您已经知道您需要一个 UNet 模型和一个调度器。
如您所见,这已经比仅包含 UNet 模型的 DDPM 管道更复杂。Stable Diffusion 模型有三个独立的预训练模型。
💡 阅读 Stable Diffusion 如何工作? 博客,以了解更多关于 VAE、UNet 和文本编码器模型如何工作的详细信息。
现在您知道了 Stable Diffusion 管道需要什么,使用 from_pretrained() 方法加载所有这些组件。您可以在预训练的 runwayml/stable-diffusion-v1-5
检查点中找到它们,每个组件都存储在单独的子文件夹中
>>> from PIL import Image
>>> import torch
>>> from transformers import CLIPTextModel, CLIPTokenizer
>>> from diffusers import AutoencoderKL, UNet2DConditionModel, PNDMScheduler
>>> vae = AutoencoderKL.from_pretrained("CompVis/stable-diffusion-v1-4", subfolder="vae", use_safetensors=True)
>>> tokenizer = CLIPTokenizer.from_pretrained("CompVis/stable-diffusion-v1-4", subfolder="tokenizer")
>>> text_encoder = CLIPTextModel.from_pretrained(
... "CompVis/stable-diffusion-v1-4", subfolder="text_encoder", use_safetensors=True
... )
>>> unet = UNet2DConditionModel.from_pretrained(
... "CompVis/stable-diffusion-v1-4", subfolder="unet", use_safetensors=True
... )
用 UniPCMultistepScheduler 代替默认的 PNDMScheduler,看看插入不同的调度器是多么容易
>>> from diffusers import UniPCMultistepScheduler
>>> scheduler = UniPCMultistepScheduler.from_pretrained("CompVis/stable-diffusion-v1-4", subfolder="scheduler")
为了加快推理速度,将模型移至 GPU,因为与调度器不同,它们具有可训练权重
>>> torch_device = "cuda"
>>> vae.to(torch_device)
>>> text_encoder.to(torch_device)
>>> unet.to(torch_device)
创建文本嵌入
下一步是标记文本以生成嵌入。文本用于对 UNet 模型进行条件化,并引导扩散过程朝向类似于输入提示的内容。
💡 guidance_scale
参数决定在生成图像时应赋予提示多少权重。
如果您想生成其他内容,请随意选择您喜欢的任何提示!
>>> prompt = ["a photograph of an astronaut riding a horse"]
>>> height = 512 # default height of Stable Diffusion
>>> width = 512 # default width of Stable Diffusion
>>> num_inference_steps = 25 # Number of denoising steps
>>> guidance_scale = 7.5 # Scale for classifier-free guidance
>>> generator = torch.manual_seed(0) # Seed generator to create the initial latent noise
>>> batch_size = len(prompt)
标记文本并从提示生成嵌入
>>> text_input = tokenizer(
... prompt, padding="max_length", max_length=tokenizer.model_max_length, truncation=True, return_tensors="pt"
... )
>>> with torch.no_grad():
... text_embeddings = text_encoder(text_input.input_ids.to(torch_device))[0]
您还需要生成无条件文本嵌入,它们是填充标记的嵌入。这些需要与条件text_embeddings
具有相同的形状(batch_size
和 seq_length
)
>>> max_length = text_input.input_ids.shape[-1]
>>> uncond_input = tokenizer([""] * batch_size, padding="max_length", max_length=max_length, return_tensors="pt")
>>> uncond_embeddings = text_encoder(uncond_input.input_ids.to(torch_device))[0]
让我们将条件和无条件嵌入连接到一个批次中,以避免执行两次前向传递
>>> text_embeddings = torch.cat([uncond_embeddings, text_embeddings])
创建随机噪声
接下来,生成一些初始随机噪声作为扩散过程的起点。这是图像的潜在表示,它将逐渐去噪。此时,latent
图像小于最终图像大小,但这没关系,因为模型稍后会将其转换为最终的 512x512 图像尺寸。
💡 高度和宽度除以 8,因为vae
模型有 3 个下采样层。您可以通过运行以下命令进行检查
2 ** (len(vae.config.block_out_channels) - 1) == 8
>>> latents = torch.randn(
... (batch_size, unet.config.in_channels, height // 8, width // 8),
... generator=generator,
... device=torch_device,
... )
对图像进行降噪
首先,使用初始噪声分布sigma(噪声尺度值)对输入进行缩放,这是改进调度器(如UniPCMultistepScheduler)所需的。
>>> latents = latents * scheduler.init_noise_sigma
最后一步是创建降噪循环,它将逐步将latents
中的纯噪声转换为您的提示描述的图像。请记住,降噪循环需要执行三件事
- 设置调度器在降噪过程中使用的时步。
- 迭代这些时步。
- 在每个时步,调用 UNet 模型来预测噪声残差,并将其传递给调度器以计算之前的噪声样本。
>>> from tqdm.auto import tqdm
>>> scheduler.set_timesteps(num_inference_steps)
>>> for t in tqdm(scheduler.timesteps):
... # expand the latents if we are doing classifier-free guidance to avoid doing two forward passes.
... latent_model_input = torch.cat([latents] * 2)
... latent_model_input = scheduler.scale_model_input(latent_model_input, timestep=t)
... # predict the noise residual
... with torch.no_grad():
... noise_pred = unet(latent_model_input, t, encoder_hidden_states=text_embeddings).sample
... # perform guidance
... noise_pred_uncond, noise_pred_text = noise_pred.chunk(2)
... noise_pred = noise_pred_uncond + guidance_scale * (noise_pred_text - noise_pred_uncond)
... # compute the previous noisy sample x_t -> x_t-1
... latents = scheduler.step(noise_pred, t, latents).prev_sample
解码图像
最后一步是使用vae
将潜在表示解码为图像,并使用sample
获取解码后的输出。
# scale and decode the image latents with vae
latents = 1 / 0.18215 * latents
with torch.no_grad():
image = vae.decode(latents).sample
最后,将图像转换为PIL.Image
以查看生成的图像!
>>> image = (image / 2 + 0.5).clamp(0, 1).squeeze()
>>> image = (image.permute(1, 2, 0) * 255).to(torch.uint8).cpu().numpy()
>>> image = Image.fromarray(image)
>>> image
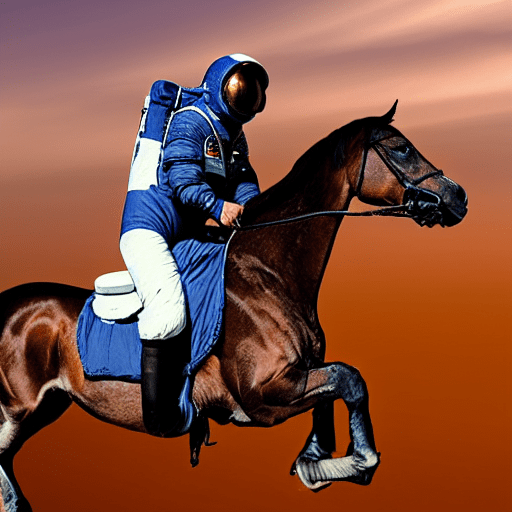
下一步
从基本的到复杂的管道,您已经看到,要编写您自己的扩散系统,您真正需要的只是一个降噪循环。该循环应该设置调度器的时步,迭代它们,并在调用 UNet 模型以预测噪声残差以及将其传递给调度器以计算之前的噪声样本之间交替进行。
这正是🧨 Diffusers 的设计目的:使您能够直观且轻松地使用模型和调度器编写您自己的扩散系统。
接下来的步骤,您可以:
< > 在 GitHub 上更新